R Create Indicator Variable
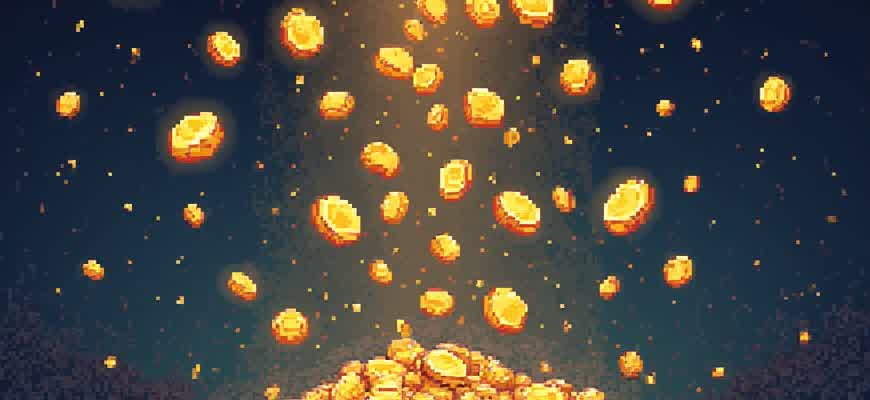
In statistical analysis, an indicator variable is a binary variable used to represent categories or groups. In R, these variables are often used for classification tasks, where they serve to indicate the presence or absence of a certain condition or characteristic.
To create an indicator variable in R, the most common approach is through conditional statements or the use of built-in functions. Below is an example of how you can create a simple indicator variable based on a categorical variable.
- Start by loading the dataset into R.
- Next, identify the categorical variable that will be converted into an indicator variable.
- Use the ifelse function to create the binary values (0 or 1) based on specific conditions.
For example, if we have a dataset with a variable Gender and we want to create an indicator variable where "Male" is represented as 1 and "Female" as 0, the code would look like this:
gender_indicator <- ifelse(data$Gender == "Male", 1, 0)
The result will be a new variable, gender_indicator, where males are assigned a value of 1 and females are assigned 0.
Note: Indicator variables are particularly useful in regression analysis, as they allow categorical data to be included in models that require numerical input.
In more complex cases, where multiple categories exist, the model.matrix function can be used to generate indicator variables for each level of the categorical variable. This results in a set of binary columns, one for each category.
- Use the model.matrix function with the formula syntax to create dummy variables for multiple categories.
- Each column will represent one category, with 1 indicating the presence and 0 indicating the absence.
Here is an example of creating indicator variables for the Region variable:
indicator_matrix <- model.matrix(~ Region - 1, data = dataset)
This will generate a matrix with columns corresponding to the different regions in the dataset.
Region | Indicator for North | Indicator for South |
---|---|---|
North | 1 | 0 |
South | 0 | 1 |
What is an Indicator Variable and How Does It Improve Data Representation?
In data analysis, an indicator variable is used to represent categorical data in a binary format, typically taking the value of 0 or 1. These variables act as flags, distinguishing between different categories or conditions. For instance, if we are examining whether an individual belongs to a particular group, the indicator variable would assign a 1 for membership and a 0 for non-membership. This transformation makes it easier for statistical models to interpret the data and perform calculations.
By converting categorical data into a numeric format, indicator variables enhance the clarity and usability of data. This approach is particularly useful when working with machine learning algorithms or regression models, which often require numerical input. Instead of treating categorical data as a single group, each category is treated as a separate binary feature, providing more granularity to the analysis.
Benefits of Using Indicator Variables
- Improved model performance: Indicator variables allow algorithms to process categorical data more efficiently, leading to better predictive accuracy.
- Clearer insights: Breaking down categorical variables into binary features makes it easier to identify patterns and relationships in the data.
- Flexibility: They allow for the inclusion of non-numeric data in various types of analyses, such as regression, classification, or clustering models.
Example of Indicator Variables
"By transforming the categorical data into binary indicators, we can better capture the nuances of each category and improve our model's predictive power."
- Consider a dataset containing information about a person's employment status: "Employed", "Unemployed", and "Student".
- We can create three indicator variables:
- Employed: 1 if employed, 0 otherwise
- Unemployed: 1 if unemployed, 0 otherwise
- Student: 1 if a student, 0 otherwise
- This allows each person's status to be captured by three separate binary features.
Indicator Variables in Practice
Person | Employed | Unemployed | Student |
---|---|---|---|
Alice | 1 | 0 | 0 |
Bob | 0 | 1 | 0 |
Charlie | 0 | 0 | 1 |
Creating Indicator Variables in R: A Step-by-Step Guide
Indicator variables, also known as dummy variables, are crucial in statistical modeling as they allow categorical data to be included in regression models. In R, these variables are typically created when working with factors or categorical variables, which need to be converted into a binary format for analysis. This guide will walk you through the process of creating indicator variables in R using simple and effective methods.
By converting categorical data into indicator variables, you can represent different categories with binary values (0 or 1). In this tutorial, we will use R's built-in functions and packages such as `model.matrix()` and `dplyr` to achieve this. Below, we outline the steps to create indicator variables from a categorical variable in R.
Step-by-Step Process
- Step 1: Load the necessary libraries.
- Step 2: Prepare your data.
- Step 3: Create indicator variables using
model.matrix()
. - Step 4: Inspect the result.
In R, you might need the `dplyr` package for data manipulation. Use the following code to load it:
library(dplyr)
Ensure that your categorical variable is in factor format. If it’s not, convert it using the factor()
function:
data$category <- factor(data$category)
This function automatically generates indicator variables for each level of the factor.
indicator_vars <- model.matrix(~ category - 1, data)
The output will be a matrix of binary values corresponding to each category. You can add these as new columns in your dataset:
data <- cbind(data, indicator_vars)
Example of Indicator Variables
Category | Indicator 1 | Indicator 2 | Indicator 3 |
---|---|---|---|
A | 1 | 0 | 0 |
B | 0 | 1 | 0 |
C | 0 | 0 | 1 |
Note: When you use model.matrix()
, the function automatically drops one level to avoid multicollinearity. This level becomes the reference category.
Common Pitfalls When Creating Indicator Variables in R
Creating indicator variables in R can be a powerful tool for transforming categorical data into numerical representations. However, this process is prone to several common errors that can impact the validity of your analysis. Below are some frequent mistakes to watch out for when generating indicator variables in R.
One of the key issues is improperly handling missing data. Indicator variables are typically created based on specific categories or conditions. If missing values are not managed properly, they can interfere with the creation process, leading to misleading results. Additionally, not using the correct reference category or neglecting factor levels can cause confusion and result in erroneous data transformations.
1. Not Handling Missing Data
Omitting or incorrectly coding missing values in your dataset can result in incorrect indicator variables. Missing data can distort the distribution and representation of the variables. Ensure that missing values are either excluded or appropriately marked as a separate category before creating indicator variables.
Tip: Always check for NA values and decide how to handle them before proceeding with the creation of indicator variables.
2. Incorrect Reference Category
When converting categorical variables into indicator variables, it is crucial to define a proper reference category. Failing to set the correct reference level can lead to inaccurate comparisons, as the wrong category may be omitted or misrepresented.
- Always double-check the factor levels before creating indicators.
- Ensure that the reference category is consistent with the context of your analysis.
3. Misunderstanding Factor Levels and Coding
R’s handling of factor variables can sometimes cause confusion, especially when the levels are not in the desired order. Misordered factor levels can lead to incorrect binary indicator values for categories. It is important to reorder factor levels before transforming them into indicators.
Incorrect Order | Correct Order |
---|---|
Low, Medium, High | Low, High, Medium |
Best Practices for Naming and Organizing Indicator Variables in R
When creating indicator variables in R, it's important to follow consistent naming conventions and maintain a logical structure. Indicator variables, often referred to as dummy variables, are binary variables used to represent categorical data. The key to managing these variables effectively is ensuring clarity, both in terms of their purpose and organization in the dataset. In this section, we will discuss best practices that help in naming and organizing these variables to improve code readability and data management.
Proper naming conventions not only enhance the code's maintainability but also prevent confusion when the dataset is shared or used for further analysis. Organizing indicator variables into logical groups ensures that the data is clean and easy to interpret. Let’s explore the essential practices for creating and managing these variables.
1. Naming Conventions for Indicator Variables
Effective naming of indicator variables should reflect the categorical feature they represent. Here are a few guidelines to follow:
- Use Descriptive Names: Names should indicate the original variable and its category. For example, if a dataset contains a "gender" variable with values "male" and "female", name the indicator variables gender_male and gender_female.
- Avoid Abbreviations: While abbreviations may seem convenient, they can create confusion later. It’s better to use full words for clarity.
- Consistency: Ensure consistent naming conventions across all indicator variables. For instance, if you choose to use underscores (_) to separate words, stick to that format throughout the dataset.
2. Organizing Indicator Variables
Organizing indicator variables in a way that groups similar categories together is essential for readability and ease of analysis.
- Keep Related Variables Together: When possible, store indicator variables for a particular categorical variable in adjacent columns to make them easy to locate.
- Group by Categories: For multi-level categorical variables, create a set of indicator variables for each level. For instance, if a variable has three categories, create three indicator variables, each representing one of the categories.
- Use Logical Ordering: Arrange indicator variables in a logical order, such as alphabetically or based on the levels of the factor variable they represent.
3. Example of Organizing Indicator Variables
Original Variable | Indicator Variable Names |
---|---|
Gender | gender_male, gender_female |
Region | region_north, region_south, region_east, region_west |
Education Level | education_highschool, education_bachelor, education_master |
Remember, the goal is to make your dataset as intuitive as possible. Clear names and logical structure will save time in the long run and make your analysis easier to follow.
How Indicator Variables Enhance Machine Learning Models in R
Indicator variables, often referred to as dummy variables, are a fundamental technique used in machine learning models. In the context of R, these variables transform categorical data into a numeric format, making it interpretable for machine learning algorithms. By converting categories into binary values (0 or 1), models can better understand and use categorical features in predictive tasks. This transformation ensures that models like linear regression, decision trees, and neural networks can efficiently process categorical data without losing information.
These variables not only help in simplifying the data preprocessing steps but also significantly improve the model’s performance. For example, when working with models like logistic regression, indicator variables can highlight the presence or absence of specific categories, aiding in better decision-making. This is especially useful when categorical variables have non-ordinal relationships, such as country names, product types, or colors, where there is no inherent ordering.
Benefits of Using Indicator Variables in R Models
- Handling Non-Numeric Data: Indicator variables convert non-numeric data into a form that can be processed by most machine learning models.
- Reducing Bias: They help in mitigating the bias that may arise from treating categorical data as continuous values.
- Improved Model Interpretability: By clearly distinguishing between different categories, indicator variables make it easier to interpret the model’s predictions.
Creating Indicator Variables in R
In R, the model.matrix() function or the fastDummies package can be used to create indicator variables efficiently. Here’s a simple example of how it can be done:
# Using model.matrix() function data <- data.frame(Category = c("A", "B", "A", "C")) dummy_vars <- model.matrix(~ Category - 1, data)
This will generate binary columns for each category in the "Category" column.
Example of Indicator Variables in a Dataset
Original Category | Indicator for A | Indicator for B | Indicator for C |
---|---|---|---|
A | 1 | 0 | 0 |
B | 0 | 1 | 0 |
A | 1 | 0 | 0 |
C | 0 | 0 | 1 |
Note: Indicator variables are especially crucial in cases where categorical data has no natural order, ensuring the model does not infer any unintended relationships.
How to Handle Missing Values When Creating Indicator Variables
When building indicator variables, missing values often pose a challenge, especially when the absence of data could influence the analysis. Handling missing values properly is crucial to ensure that the newly created indicator variables are accurate and do not introduce biases into your model. The method used to deal with missing data should be carefully selected based on the nature of the dataset and the analytical goals.
There are several strategies for addressing missing values in the context of creating indicator variables. Some methods fill in the missing values with a placeholder, while others might exclude rows with missing data altogether. The choice depends on the type of missingness and the desired outcome in the analysis.
Approaches to Handling Missing Values
- Omission of Rows: One straightforward method is to remove any rows with missing values before creating the indicator variable. This approach works well if the number of missing entries is small and does not significantly impact the dataset.
- Imputation: In some cases, filling missing values with the mean, median, or mode of the non-missing entries may be appropriate. This ensures that all observations are included in the dataset while minimizing bias.
- Indicator for Missingness: Another option is to create a separate indicator variable specifically for missing data. This new variable would take the value of 1 when data is missing and 0 when data is present.
Steps to Create Indicator Variables for Missing Data
- Examine the data to determine the pattern of missingness (e.g., missing completely at random, missing at random, missing not at random).
- Decide on the handling method: whether to omit the rows, impute values, or create an indicator variable.
- If creating an indicator variable, use a logical condition to assign 1 for missing and 0 for present, ensuring consistency across the dataset.
Important: Always consider the impact of missing data on the analysis. Simply omitting rows with missing values can reduce the dataset size, while imputing data could introduce inaccuracies if not done carefully.
Example of an Indicator Variable for Missing Data
Original Data | Indicator for Missing |
---|---|
25 | 0 |
NA | 1 |
45 | 0 |
NA | 1 |
Optimizing Data Size and Performance When Working with Indicator Variables in R
When working with indicator variables in R, efficiency is key to ensuring that your data processing remains fast and scalable. Indicator variables, which represent binary states (such as 1 for "Yes" and 0 for "No"), are commonly used to encode categorical information. While this approach is intuitive and convenient, it can lead to large datasets and performance issues, particularly when dealing with large volumes of data. It's essential to adopt strategies that can minimize memory usage and enhance computational speed without compromising the integrity of the data.
One effective way to optimize performance is by reducing the number of indicator variables. Instead of creating separate variables for every category in a dataset, consider using encoding techniques such as factor variables or dummy coding, which allow for more efficient data storage. Another technique is to apply sparse matrices, which store only non-zero values, saving memory and computational resources. Below are a few strategies to ensure that your code performs efficiently when dealing with indicator variables:
Techniques for Optimizing Data with Indicator Variables
- Use Factor Variables: R provides a factor class that stores categorical data more efficiently than character vectors. By converting categorical columns to factors, memory usage is reduced, and performance is enhanced.
- Sparse Matrices: When working with a large number of indicator variables, consider using sparse matrices to store only the non-zero elements. This can significantly reduce memory consumption.
- Remove Unnecessary Variables: After creating indicator variables, assess whether they are still needed in your dataset. Removing redundant variables can free up memory and speed up processing.
- Data Compression: Use R’s data compression capabilities, such as saving data frames in compressed formats, to reduce file sizes while maintaining accessibility.
Best Practices for Efficient Performance
- Preprocess data to combine categories with small frequencies, which reduces the number of indicator variables required.
- Use efficient data structures like data.table instead of data.frame for large datasets to speed up operations on indicator variables.
- Take advantage of vectorized operations in R, which are faster than loops for manipulating indicator variables.
Example of Efficient Use of Indicator Variables
Category | Original Encoding | Optimized Encoding (Factor) |
---|---|---|
Category A | 1 | Factor(1) |
Category B | 0 | Factor(0) |
Category C | 1 | Factor(1) |
By efficiently encoding categorical data as factors instead of using multiple indicator variables, you can dramatically reduce the size of your dataset, leading to faster performance in data processing tasks.
Integrating Indicator Variables with Other R Functions for Advanced Analysis
Indicator variables are essential in data analysis, particularly when working with categorical data or performing regression analysis. These variables allow for the representation of categories numerically, enabling statistical models to process non-numeric data. However, simply creating indicator variables is not enough–incorporating them effectively into other R functions can significantly enhance your analysis capabilities. This can be achieved through techniques such as subsetting, merging, or interaction with other functions like model fitting and visualization tools.
To maximize the utility of indicator variables, it's crucial to integrate them with various R functions, such as those used in data manipulation, modeling, and visualization. This integration is what leads to more insightful and nuanced analyses, especially when handling large datasets with multiple categories or factors. Here, we'll look at a few methods for integrating indicator variables with other functions in R to achieve advanced analyses.
Using Indicator Variables with Data Manipulation Functions
One of the primary ways to integrate indicator variables into your analysis is through data manipulation techniques. Functions from the dplyr and tidyr packages in R can help you filter, arrange, and transform your data, while keeping the indicator variables intact. For example, you might use an indicator variable to filter observations belonging to a particular group.
- filter() - Filter data based on indicator variables to create subsets for further analysis.
- mutate() - Add new indicator variables to the dataset based on conditions.
- spread() - Reshape data using indicator variables to create wide-format datasets.
Incorporating Indicator Variables into Regression Models
Indicator variables are often used as factors in regression models, where they help capture the effect of categorical variables on the outcome. Functions like lm() and glm() in R make it simple to incorporate indicator variables for modeling purposes.
- lm() - Used for linear models where indicator variables serve as explanatory variables.
- glm() - Used for generalized linear models, allowing the inclusion of indicator variables as predictors.
When using indicator variables in regression, ensure that you do not include a reference category, as it may cause multicollinearity issues.
Example: Indicator Variables in a Regression Model
Variable | Type | Description |
---|---|---|
Age | Continuous | Age of the individual |
Gender | Indicator | 1 for male, 0 for female |
Income | Continuous | Annual income of the individual |
In this case, the Gender variable is an indicator variable that can be used to analyze its effect on Income, controlling for the variable Age.