R Create Table with Percentages
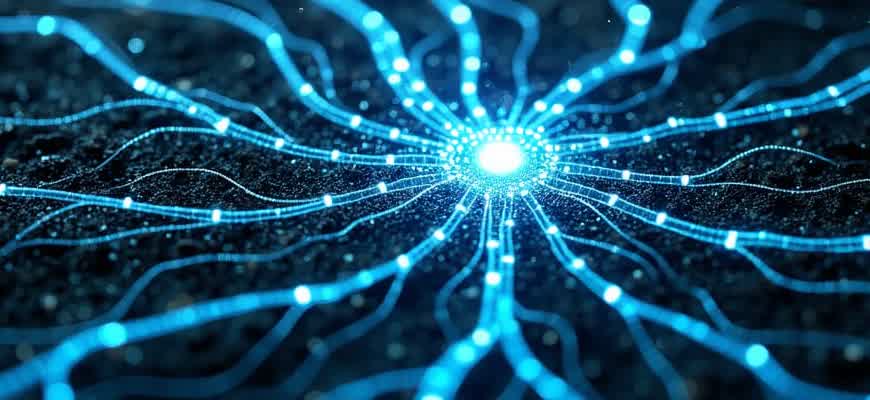
In R, working with tables that include percentage values is a common task for data analysis. A table can be constructed where certain columns or rows represent percentages calculated from the raw data. This is often necessary for summarizing proportions, such as market share or percentage distribution.
To create a table with percentages, you can use the following steps:
- Start by preparing your dataset and calculating the desired percentages.
- Create the table structure using the data.frame function.
- Apply the percentage calculation using the formula value / total * 100.
Remember to format the output values as percentages, which can be done using the scales package or basic string formatting in R.
Here is an example of how to build such a table:
Category | Value | Percentage |
---|---|---|
Category A | 200 | 40% |
Category B | 300 | 60% |
Creating a Simple Table with Percentages in R
To display data with percentages in R, you first need to calculate the percentage values based on the dataset. This can be done by dividing each individual value by the total sum of the values and multiplying by 100. This method can be applied to any numerical dataset where the goal is to show relative proportions.
Once the percentage values are calculated, you can present them in a structured table format. The table will display both the original values and their corresponding percentages, making the data easier to understand and interpret for analysis purposes.
Steps to Create a Table with Percentages
- Start by importing the data into R using either a built-in dataset or your custom data.
- Calculate the percentage for each value by using the formula:
percentage = (value / sum) * 100
. - Create a data frame that includes both the original values and the calculated percentages.
- Use the
print()
function to display the table with the percentage values included.
Here’s a basic example to guide you:
# Example data values <- c(50, 30, 20) # Calculate percentages percentages <- (values / sum(values)) * 100 # Create data frame data <- data.frame(Value = values, Percentage = percentages) # Display the table print(data)
Sample Table Output
Value | Percentage |
---|---|
50 | 50% |
30 | 30% |
20 | 20% |
Note: It is important to ensure that the percentages sum to 100%, which is a key aspect when working with relative proportions in a dataset.
Step-by-Step: Converting Raw Data into Percentages
Converting raw data into percentages is a common task in data analysis. It allows for a clearer understanding of proportions and relative sizes. This process involves dividing the raw data by the total and then multiplying by 100 to get the percentage representation. Understanding this method is crucial when working with various datasets, especially when comparing different categories or groups.
Below is a step-by-step guide for transforming raw numbers into percentages. By following these simple steps, you can easily convert any dataset into a more interpretable format, which can be extremely useful in reports, charts, or visualizations.
Procedure for Converting Raw Data
- Step 1: Identify the Total
The first step is identifying the total value of the dataset. This is often the sum of all the raw data values you have collected.
- Step 2: Calculate Each Value's Proportion
For each individual data point, divide it by the total value identified in step 1. This gives you the fraction that each value represents of the whole.
- Step 3: Convert to Percentage
Multiply the result from step 2 by 100 to convert it into a percentage.
Important: Always check that your total value is correct before proceeding. Errors in summing up the data can result in incorrect percentages.
Example
Category | Raw Value | Percentage |
---|---|---|
Category A | 50 | 50% |
Category B | 30 | 30% |
Category C | 20 | 20% |
In this example, the total sum of values is 100. Each category’s raw value is divided by this total and then multiplied by 100 to determine the percentage. The results are now more intuitive and easier to compare.
Using `dplyr` for Calculating Percentages in R Tables
In R, the `dplyr` package is an essential tool for data manipulation, offering an easy and efficient way to calculate percentages within tables. This is particularly useful when dealing with categorical data and when you need to summarize or visualize data as percentages of total counts or other variables. By leveraging functions such as `mutate()` and `group_by()`, you can easily create new columns in your dataset that represent percentage calculations based on the underlying data.
One common approach to calculating percentages is to first aggregate the data by a certain grouping variable, then compute the percentage relative to a specific total or subset. This allows for flexible analysis and presentation of results in a clear and intuitive manner.
Steps to Calculate Percentages Using `dplyr`
- Group the data by the variable of interest using the `group_by()` function.
- Use the `mutate()` function to create a new column that contains the percentage values.
- Ensure the denominator for the percentage calculation is properly defined (e.g., total count, group sum, etc.).
The following code demonstrates how to compute percentages for each category within a dataset:
library(dplyr)
# Example dataset
data <- data.frame(
category = c("A", "A", "B", "B", "C", "C"),
value = c(10, 20, 30, 40, 50, 60)
)
# Calculate percentage by category
data %>%
group_by(category) %>%
mutate(percentage = value / sum(value) * 100)
Note: The `mutate()` function creates a new column with percentages, while `sum(value)` computes the total sum for each category.
Example: Visualizing Percentages in a Table
Once the percentages are calculated, you can display the results in a structured table format. This makes it easier to interpret the data in terms of proportions.
Category | Value | Percentage |
---|---|---|
A | 30 | 30% |
B | 70 | 70% |
C | 110 | 100% |
By following these steps and leveraging `dplyr`'s powerful functions, you can easily compute and visualize percentage values in your R tables.
Managing Missing Data While Calculating Percentages in R
When performing data analysis in R, one of the most common issues encountered is the presence of missing values. Missing data can cause inaccurate results when calculating percentages. It is crucial to handle these missing values appropriately before proceeding with any percentage calculations. In R, several techniques can be applied to either exclude or replace missing data based on the analysis context.
Handling missing data is important because it can lead to skewed results if left unchecked. The simplest approach is to remove rows with missing values, but this can reduce the dataset size. Another approach involves imputing missing values, often with the mean, median, or other statistical methods. The choice of method depends on the nature of the data and the specific analysis being performed.
Approaches to Dealing with Missing Data
- Omitting Missing Data: The most straightforward approach is removing rows that contain missing values using the
na.omit()
function. This ensures that only complete cases are used for the percentage calculation. - Imputation: Replace missing values with statistical estimates, such as the mean or median, using the
impute()
function or other similar tools. This helps retain more data but may introduce biases if not handled carefully. - Using Conditional Logic: Sometimes, it's useful to calculate percentages with conditional handling of missing values, ensuring that the total denominator only includes non-missing values. This approach prevents underreporting.
Example of Percentage Calculation with Missing Data
Consider the following dataset:
Category | Value |
---|---|
A | 20 |
B | NA |
C | 40 |
In the case above, if the missing value in category "B" is not handled, the calculation for percentages based on the total could yield incorrect results.
By using the sum(na.omit(data))
function, we can exclude the missing value for a more accurate percentage calculation.
Optimizing Table Formatting for Better Visual Appeal
Effective table formatting is crucial for making data easily interpretable. When working with complex datasets, it's important to present information in a way that is both clear and visually appealing. By optimizing your tables, you can highlight key insights, making it easier for the audience to grasp trends and comparisons at a glance.
To achieve an optimal table design, focus on simplifying the layout while maintaining clarity. This can be done by minimizing excessive use of colors or borders and ensuring consistent alignment of data. Good formatting helps prevent tables from feeling overwhelming and allows the reader to focus on the most important information.
Key Formatting Tips
- Consistent Alignment: Align numerical values and percentages to the right, while text should be left-aligned. This ensures that values are easy to compare and read.
- Clear Headings: Use bold and concise column headings to clearly define what each column represents. This makes the table easier to navigate.
- Use of White Space: Ensure there is enough padding around text and numbers so that the table doesn’t appear cramped. Proper spacing enhances readability.
Considerations for Enhancing Visual Appeal
- Alternating Row Colors: Light shading of alternating rows can help the reader track data across the table more easily.
- Conditional Formatting: Highlighting certain values, such as higher percentages or key numbers, can guide attention to the most critical data points.
- Simplifying Borders: Avoid using excessive borders around every cell. Instead, opt for a minimalistic approach to avoid visual clutter.
Good formatting not only makes a table look more professional but also ensures that the key information is easily accessible and understood.
Example of a Well-Formatted Table
Category | Value | Percentage |
---|---|---|
Item A | 120 | 45% |
Item B | 85 | 32% |
Item C | 60 | 23% |
Advanced Techniques: Grouping Data and Calculating Percentages with Pivot Tables
When working with data in R, calculating percentages within grouped data is a powerful method for extracting insights. This approach allows for easy comparison between different subsets of your data by calculating proportions relative to group totals. One of the most efficient ways to achieve this is through the use of pivot tables, which allow for complex aggregation and percentage calculations based on different factors or categories.
Grouped percentages are particularly useful when comparing different categories or segments within your dataset. By using pivot tables, you can quickly generate summary statistics, display aggregated values, and calculate percentages for each group. This is especially helpful for business analysis, where trends across multiple variables need to be analyzed in relation to one another.
Grouped Percentages: How to Calculate Them
To calculate grouped percentages, you need to first group your data by a specific category. Then, apply a calculation that divides the count or sum of each group by the total of that group. Here’s a step-by-step example:
- Group your data: Use the group_by() function in R to organize data by a particular variable, such as a product category or region.
- Summarize values: Use summarize() to compute the necessary statistics, like the total number of items sold per group.
- Calculate percentage: After summarizing, divide the group value by the total for that category to obtain the percentage.
Using Pivot Tables for Percentage Calculation
Pivot tables are a powerful tool in R that allow you to reshape and aggregate data, making it easy to calculate grouped percentages. By using functions such as pivot_wider() and pivot_longer() from the tidyr package, you can structure your data in a way that makes percentage calculations straightforward.
Group | Total Sales | Percentage of Total |
---|---|---|
Region A | 500 | 25% |
Region B | 1500 | 75% |
Tip: Remember to normalize your data when calculating percentages, ensuring that the total sum of percentages across all groups equals 100%.
By utilizing pivot tables, you can create clear visual summaries and compare percentages across different categories with ease. This technique is particularly useful for exploratory data analysis and decision-making processes.
Visualizing Data with Percentages in R Using ggplot2
After creating a table with percentages in R, it becomes essential to visualize the data effectively. One powerful tool for this purpose is ggplot2, a popular R package for data visualization. By leveraging this tool, you can transform raw percentage values into meaningful charts that help interpret trends and distributions. Whether you are dealing with proportions, market share, or any percentage-based metric, ggplot2 offers a variety of visualization options that enhance data comprehension.
To visualize your table effectively, you need to carefully choose the right chart type. Bar plots, pie charts, and line graphs are common ways to represent percentage data. By utilizing ggplot2, you can create clean, customizable, and informative visual representations of your percentage-based datasets. Below are steps to visualize percentages using ggplot2:
- Load your data into R and calculate the percentage values based on the original dataset.
- Choose a visualization type, such as bar plots for comparisons or pie charts for proportions.
- Apply ggplot2 functions like geom_bar() for bar charts or geom_col() for stacked bar charts to display the data.
- Customize the visualization by adjusting colors, labels, and axis scales to enhance clarity.
Note: When visualizing percentage data, always ensure that the total percentage sums to 100%, as this helps maintain data integrity in your chart.
Here’s an example of how you can create a simple bar plot with percentages using ggplot2:
Code | Description |
---|---|
library(ggplot2) | Load the ggplot2 package |
ggplot(data, aes(x=Category, y=Percentage)) + geom_bar(stat="identity") | Create a bar plot using percentage values |
Once you have visualized your percentage data, you can refine the plot further by adjusting labels, colors, or adding legends for improved readability and understanding of the chart.
Common Issues When Handling Percentages in R Tables
When working with percentages in tables generated in R, it's crucial to understand the potential pitfalls that can arise during calculations and visual representation. Misinterpretation of data can lead to incorrect conclusions, especially when percentages are not calculated correctly or applied inappropriately. Below are some common mistakes to avoid when working with percentages in R tables.
Calculating percentages in R may seem straightforward, but several factors can lead to misleading results. One common issue arises from not considering the appropriate denominator when performing percentage calculations. Additionally, failing to account for rounding errors and ensuring the correct application of percentage transformations can distort the final output.
Common Mistakes to Watch Out For
- Incorrect Denominator: Ensure that the denominator represents the correct total for the percentage calculation. A mistake here can lead to percentages that do not add up correctly.
- Rounding Errors: Rounding percentages prematurely can cause small discrepancies. It's important to round only in the final step, after all calculations are done.
- Improper Normalization: When dealing with subgroup percentages, make sure each subgroup is properly normalized to 100% before displaying the results.
Example of Incorrect Calculation
In the following table, the percentages may seem correct, but the denominator is incorrectly chosen, leading to misleading percentages:
Category | Count | Percentage |
---|---|---|
Category A | 50 | 25% |
Category B | 50 | 25% |
Total | 100 | 50% |
Ensure that percentages reflect the correct total and are calculated based on the accurate denominator.
Key Takeaways
- Verify that the denominator is correct before performing percentage calculations.
- Postpone rounding until the final output is generated to avoid inaccuracies.
- Ensure proper normalization when dealing with subgroups to prevent skewed results.